Location Service APIs to solve vehicle routing problems
PTV Developer offers high-performance APIs that enrich your software solution by logistical and geographical functionalities. The APIs can be combined and customized as needed and scale easily. Integrate PTV Developer APIs to boost the efficiency and performance of your logistics and to give your business a competitive edge.
OptiFlow route optimization algorithms now available as API
NEW and available now – Integrate our superior & high-performance route optimization algorithms directly into your platform and enhance it with cutting-edge technology designed for developers, by developers. The Route Optimization OptiFlow API can be tested for free!
Customers who are happy with our APIs
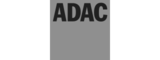
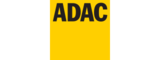
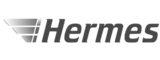
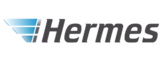
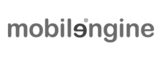
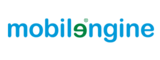
Reasons why you should use the PTV Developer APIs
Integrate the functionalities into your system environment easily and seamlessly
Select and combine the applications you need and add additional ones at any time
Consider real-life constraints, find solutions to everyday problems, and improve communication
PTV Developer APIs - State-of-the-art cloud technology
PTV Developer offers performant state-of-the-art APIs that solve logistical and geographical challenges. We provide an OpenAPI standard, which allows for seamless integration into web-based applications based on modern RESTful APIs. PTV Developer’s reliability is unmatched: We ensure that systems are up and running at least 99.9% of the time.
Depending on your challenges, you can select the APIs you need and adapt them to your individual requirements. When your business grows or your needs change, you can easily add functionalities and scale your solution accordingly. As all data is stored and remains within the European Union, you can rest assured that the use of PTV Developer is absolutely GDPR compliant.
Here's an overview of the APIs we offer:
Geocoding & Places API
Find geolocations, convert street addresses into GPS coordinates, and reverse geocode locations.
Geocoding & Places OSM API
Accurately convert postal addresses to GPS coordinates based on OpenStreetMap. Easily enter addresses using any format. Find landmarks and addresses based on their GPS coordinates.
Vector Maps OSM API
Render geographical vector tiles based on OpenStreetMap with easily integrable open-source frameworks.
Routing API
Plan routes that save time and money for any vehicle, while providing accurate arrival times.
Routing OSM API
Plan routes that save time and money for bicycles, cars and trucks. It is built on OpenStreetMap (OSM) data.
Matrix Routing API
Calculate distances and travel times between multiple locations quickly and accurately.
Matrix Routing OSM API
Calculate distances and travel times between multiple locations quickly and accurately based on OpenStreetMap data.
Route Optimization API
Schedule and optimize your fleet routes to ensure the most efficient use of resources.
Route Optimization OptiFlow API
Find the most efficient way to distribute goods or services to multiple destinations using a fleet of vehicles.
Sequence Optimization API
Optimize stop order to get the most efficient routes for your vehicles.
Loading Space Optimization API
Optimize cargo loadings by minimizing free volumes and considering unloading sequence.
EWS API
Use an industry standard distance matrix as a basis for tender management, invoice auditing and credit note procedures.
Get in touch
Want to learn more? Contact our experts and discover all the features and benefits of PTV Developer by filling out the contact form, or call us directly.
Phone number: +49 721 91112-8100